A sample paragraph with a data attribute.
Responsive column layout
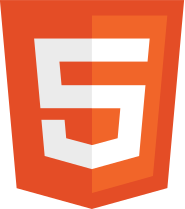
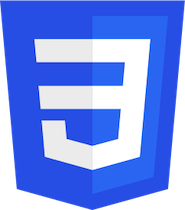

With the General HTML Support (GHS) feature, developers can enable HTML features that are not supported by any other dedicated CKEditor 5 plugins. GHS lets you add elements, attributes, classes, and styles to the source. It also ensures this markup stays in the editor window and in the output.
Use the Enhanced source code editing feature toolbar button to view and edit the HTML source of the document. You can find the configuration of this snippet below the demo.
You can configure the General HTML Support feature using the config.htmlSupport
property. With this property, you need to list the HTML features that should be handled by GHS.
This demo presents a limited set of features. Visit the feature-rich editor example to see more in action.
Here are some examples of HTML features that you can enable using General HTML Support:
<section>
, <article>
, and <div>
elements.<audio>
, <video>
, and <iframe>
elements.<span>
and <cite>
elements.data-*
and id
attributes on, for example, <p>
and <h1-h6>
.style
and classes
attributes on, for example, <strong>
and <a>
.You can load (for example, via editor.setData()
), paste, output (for example, via editor.getData()
) the enabled HTML features. They are also visible in the editing area. To a limited extent, you can also edit such content in the editor. Read more about it in the Level of support section.
The difference between specific CKEditor 5 features such as basic styles or headings and the HTML features enabled by GHS is that a plugin that supports a specific HTML feature provides a complete user experience for that feature. GHS ensures only that such content is accepted by the editor.
For instance, the dedicated bold feature offers a toolbar button used to make the selected text bold. Together with the autoformatting feature, it also allows for applying bold style to content by typing a Markdown shortcode (**foo**
) in the editor. The headings feature offers a dropdown from which the user can choose a heading level and ensures that pressing Enter at the end of a heading creates a new paragraph (and not another heading).
The General HTML Support does not offer any UI for the enabled features and takes only the basic semantics of a given feature into account. If you enable support for <div>
elements via GHS, the user will not be able to create such elements from the editor UI. The GHS will know that a <div>
is a container element, so it can wrap other blocks (like paragraphs) but cannot be used inline (next to, for example, a <strong>
element). In this respect, it is similar to the Advanced Content Filtering (ACF) feature from CKEditor 4 as it lets you create a list of markup tags that the editor will not strip.
Therefore, the main use cases for GHS would be:
Considering the nature of GHS, you may consider installing the Enhanced source code editing feature alongside it.
⚠️ New import paths
Starting with version 42.0.0, we changed the format of import paths. This guide uses the new, shorter format. Refer to the Packages in the legacy setup guide if you use an older version of CKEditor 5.
After installing the editor, add the feature to your plugin list and toolbar configuration:
import { ClassicEditor, GeneralHtmlSupport } from 'ckeditor5';
ClassicEditor
.create( document.querySelector( '#editor' ), {
licenseKey: '<YOUR_LICENSE_KEY>', // Or 'GPL'.
plugins: [ GeneralHtmlSupport, /* ... */ ],
htmlSupport: {
// Configuration.
}
} )
.then( /* ... */ )
.catch( /* ... */ );
By default, enabling the GeneralHtmlSupport
plugin does not enable support for any given element. You need to configure the elements the user wants to use via the config.htmlSupport
option:
ClassicEditor
.create( document.querySelector( '#editor' ), {
// ... Other configuration options ...
htmlSupport: {
allow: [ /* HTML features to allow. */ ],
disallow: [ /* HTML features to disallow. */ ]
}
} )
.then( /* ... */ )
.catch( /* ... */ );
The notation of the allow
and disallow
rules looks as follows:
[
{
// The element name to enable or extend with
// the following styles, classes, and other attributes.
name: string|regexp,
// Styles to allow (by name, name and value, or just all).
styles: object<string=>true|string|regexp>|array<string>|true,
// Classes to allow (by name or just all).
classes: array<string|regexp>|true,
// Other attributes to allow (by name, name and value, or just all).
attributes: object<string=>true|string|regexp>|array<string>|true,
}
]
Several implementation examples:
htmlSupport: {
allow: [
// Enables plain <div> elements.
{
name: 'div'
},
// Enables plain <div>, <section>, and <article> elements.
{
name: /^(div|section|article)$/
},
// Enables <div> elements with all inline styles (but no other attributes).
{
name: 'div',
styles: true
},
// Enables <div> elements with "foo" and "bar" classes.
{
name: 'div',
classes: [ 'foo', 'bar' ]
},
// Adds support for "foo" and "bar" classes to the already supported
// <p> elements (those are enabled by the dedicated paragraph feature).
{
name: 'p',
classes: [ 'foo', 'bar' ]
},
// Enables <div> elements with foo="true" attribute and "bar" attribute that
// can accept any value (the Boolean "true" value works as an asterisk).
{
name: 'div',
attributes: {
foo: 'true',
bar: true
}
},
// Adds support for style="color: *" to the already supported
// <p> and <h2-h4> elements.
{
name: /^(p|h[2-4])$/',
styles: { 'color': true }
},
}
The General HTML Support feature distinguishes several content types, each treated a bit differently:
<section>
, <div>
).<span>
, <a>
).<iframe>
, <video>
).The enabled elements will not just be available “anywhere” in the content. They still need to adhere to certain rules derived from the HTML schema and common sense. Also, the behavior of specific types of elements in the editing area will be different. For instance, the object elements will only be selectable as a whole. The inline elements will work the same as other formatting features supported by CKEditor 5 (like bold and italic) do.
Sometimes you might want to enable all HTML features, so the editor will allow all elements and attributes. You can achieve this with a special configuration:
htmlSupport: {
allow: [
{
name: /.*/,
attributes: true,
classes: true,
styles: true
}
]
}
Enabling all HTML features creates a security risk. You should pass a list of disallowed elements and attributes to the configuration to make sure that any malicious code will not be saved and executed in the editor.
This configuration will work similarly to the allowedContent: true
option from CKEditor 4.
When you set up the GHS to allow elements like <script>
or attributes like onclick
, you expose the users of your application to a possibly malicious markup. This can be code mistakenly copied from a risky website or purposely provided by a bad actor. An example of that could be: <div onclick="leakUserData()">
.
The content inside the editor (what you see in the editing area) is filtered by default from typical content that could break the editor. However, the editor does not feature a full XSS filter. We recommend configuring GHS to enable specific HTML markup, instead of enabling all markup at once.
Moreover, as a general rule, not exclusive to GHS, there should always be a sanitization process present on the backend side of your application. Even the best filtering done on the browser side of your application can be mitigated and every network call can be manipulated, thus bypassing the frontend filtering. This can quickly become a security risk.
In addition to the sanitization process and safe GHS configuration, it is highly recommended to set strict Content Security Policy rules.
You can define custom HTML elements with attributes and classes.
To use a new element, you need to register it with DataSchema
as one of the types below:
To enable such elements and add attributes or classes to them, you need to use the allowElement
and allowAttributes
methods from the DataFilter
API.
Base implementation example:
import { ClassicEditor, Essentials, Paragraph, Plugin, SourceEditingEnhanced, GeneralHtmlSupport } from 'ckeditor5';
/**
* A plugin extending General HTML Support, for example, with custom HTML elements.
*/
class ExtendHTMLSupport extends Plugin {
static get requires() {
return [ GeneralHtmlSupport ];
}
init() {
// Extend the schema with custom HTML elements.
const dataFilter = this.editor.plugins.get( 'DataFilter' );
const dataSchema = this.editor.plugins.get( 'DataSchema' );
// Inline element.
dataSchema.registerInlineElement( {
view: 'element-inline',
model: 'myElementInline'
} );
// Custom elements need to be registered using direct API instead of configuration.
dataFilter.allowElement( 'element-inline' );
dataFilter.allowAttributes( { name: 'element-inline', attributes: { 'data-foo': false }, classes: [ 'foo' ] } );
// Block element.
dataSchema.registerBlockElement( {
view: 'element-block',
model: 'myElementBlock',
modelSchema: {
inheritAllFrom: '$block'
}
} );
dataFilter.allowElement( 'element-block' );
}
}
ClassicEditor
.create( document.querySelector( '#editor' ), {
plugins: [
Essentials,
Paragraph,
SourceEditingEnhanced,
ExtendHTMLSupport
],
htmlSupport: {
allow: [
{
name: /.*/,
attributes: true,
classes: true,
styles: true
}
]
}
} )
.then( /* ... */ )
.catch( /* ... */ );
You can treat both inline and block elements as object elements. To make it possible, it is necessary to set the isObject property to true
.
// Inline object element.
dataSchema.registerInlineElement( {
view: 'object-inline',
model: 'myObjectInline',
isObject: true,
modelSchema: {
inheritAllFrom: '$inlineObject'
}
} );
dataFilter.allowElement( 'object-inline' );
// Block object element.
dataSchema.registerBlockElement( {
view: 'object-block',
model: 'myObjectBlock',
isObject: true,
modelSchema: {
inheritAllFrom: '$blockObject'
}
} );
dataFilter.allowElement( 'object-block' );
You can add support for arbitrary styles, classes, and other attributes to existing CKEditor 5 features (such as paragraphs, headings, list items, etc.). Most of the existing CKEditor 5 features can already be extended this way, however, some cannot yet.
While the GHS feature is stable, some problems with complex documents may occur if you use it together with real-time collaboration.
We are open to feedback, so if you find any issue, feel free to report it in the main CKEditor 5 repository.
CKEditor 5 has other features related to HTML editing that you may want to check:
<html>
to </html>
, including the page metadata.Every day, we work hard to keep our documentation complete. Have you spotted outdated information? Is something missing? Please report it via our issue tracker.
With the release of version 42.0.0, we have rewritten much of our documentation to reflect the new import paths and features. We appreciate your feedback to help us ensure its accuracy and completeness.